Table of Contents
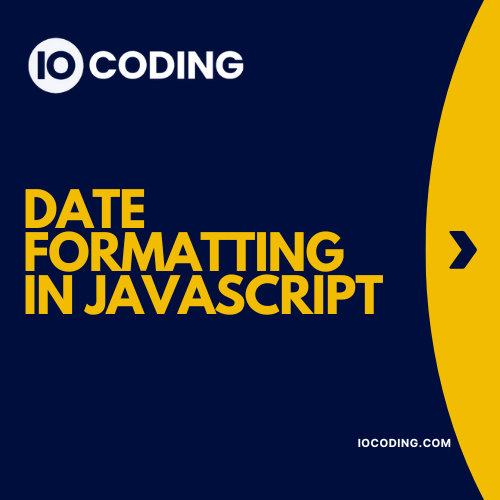
In the realm of web development, managing and displaying dates effectively is paramount. Dates are a fundamental component of numerous web applications, ranging from simple blogs to complex financial systems. Proper date formatting ensures that users can effortlessly understand and interact with the data presented. Without consistent and clear date formats, applications can become confusing and challenging to navigate, potentially leading to user frustration and errors.
The significance of date formatting extends beyond mere aesthetics. It plays a crucial role in ensuring data accuracy and maintaining consistency across different parts of an application. For instance, displaying a date in various formats depending on the user’s locale can enhance the user experience and make the application more accessible globally. Moreover, standardized date formats are essential for functions such as sorting, filtering, and validating dates.
This comprehensive guide will delve into the art of mastering date formatting in JavaScript. We will explore native JavaScript methods for handling dates, which provide basic yet powerful tools for date manipulation. Additionally, we will examine two popular libraries—date-fns and moment.js—that offer more advanced and user-friendly solutions for date formatting and manipulation. These libraries provide a wealth of functionality that can simplify and enhance date handling in your applications.
Furthermore, we will discuss best practices for date formatting to ensure that your web applications are both user-friendly and maintainable. This includes tips on choosing the right library for your needs, understanding the importance of time zones, and implementing consistent date formats across your application. By the end of this guide, you will be equipped with the knowledge and tools necessary to handle date formatting in JavaScript with confidence and precision.
Using Native JavaScript Date Methods
JavaScript provides native methods for creating and formatting dates, which are essential for everyday development tasks. The journey begins with understanding how to instantiate a new Date object. The Date object can be created using the `new Date()` constructor, which can take no arguments (defaulting to the current date and time), or specific date and time values.
Here is an example of creating a new Date object:
const now = new Date(); // Current date and time
const specificDate = new Date('2023-10-01T10:20:30Z'); // Specific date and time
Once you have a Date object, you can format it using several built-in methods. The `toDateString()` method returns the date portion of the date object as a human-readable string. This is useful when you need a quick, readable date format.
Example:
console.log(now.toDateString()); // Outputs: 'Mon Oct 01 2023'
The `toTimeString()` method, on the other hand, returns the time portion of the date object as a human-readable string. This method is particularly beneficial when you need to display only the time.
Example:
console.log(now.toTimeString()); // Outputs: '10:20:30 GMT+0000 (Coordinated Universal Time)'
For more locale-specific formatting, the `toLocaleDateString()` and `toLocaleTimeString()` methods are invaluable. These methods allow you to format dates and times according to the conventions of a specific locale, which is crucial for international applications.
Example of `toLocaleDateString()`:
console.log(now.toLocaleDateString('en-US')); // Outputs: '10/1/2023'
Example of `toLocaleTimeString()`:
console.log(now.toLocaleTimeString('en-US')); // Outputs: '10:20:30 AM'
Each method serves different purposes based on the requirement of your application. While `toDateString()` and `toTimeString()` provide quick, readable formats, `toLocaleDateString()` and `toLocaleTimeString()` offer flexibility and cater to locale-specific needs.
Formatting Dates with date-fns
When it comes to date formatting in JavaScript, date-fns is a powerful and versatile library that stands out for its simplicity and modularity. This library provides a comprehensive set of tools for manipulating dates, making it an essential choice for developers who need to handle date formatting efficiently.
Installing date-fns
To start using date-fns, you first need to install it via npm. The installation process is straightforward. Open your terminal and run the following command:
npm install date-fns
Once the installation is complete, you can begin using date-fns in your JavaScript projects.
Using date-fns to Format Dates
Formatting dates with date-fns is intuitive and easy. Here is an example to illustrate the process:
import { format } from 'date-fns';
const date = new Date();
const formattedDate = format(date, 'yyyy-MM-dd');
console.log(formattedDate); // Output: 2023-10-05
In this example, the format
function from date-fns is used to format a date object into a string with the format ‘yyyy-MM-dd’.
Popular date-fns Formats
date-fns supports a variety of date formats to cater to different needs. Some of the most popular formats include:
- yyyy-MM-dd: This format represents a date in the year-month-day sequence, such as 2023-10-05.
- MM/dd/yyyy: This format uses the month-day-year sequence, common in the United States, for example, 10/05/2023.
- dd-MM-yyyy: This format, often used in Europe and other regions, represents a date in the day-month-year sequence, such as 05-10-2023.
These formats are versatile and can be adjusted to meet various regional and contextual requirements.
Advantages of date-fns
One of the key advantages of date-fns is its modularity. Unlike some other libraries, date-fns allows you to import only the functions you need, reducing the overall bundle size and improving performance. Additionally, date-fns supports functional programming, enabling developers to write cleaner and more maintainable code. Its comprehensive documentation and active community further enhance its usability, making date-fns a preferred choice for date date formatting in JavaScript.
Formatting Dates with Moment.js
Moment.js is a widely-used JavaScript library for date manipulation and formatting, providing a vast array of functionalities to handle dates and times efficiently. To begin using Moment.js, you need to install it via npm. Open your terminal and execute the following command:
npm install moment
Once installed, Moment.js can be utilized to format dates with ease. Below is a basic example demonstrating how to format a date using Moment.js:
const moment = require('moment');
const date = moment();
console.log(date.format('YYYY-MM-DD'));
In this instance, the date is formatted in the ‘YYYY-MM-DD’ format, a common date representation. Moment.js supports a variety of date formats, some of the most popular being:
- YYYY-MM-DD: This format is widely used in databases and APIs due to its standardization and readability.
- MM/DD/YYYY: Commonly used in the United States, this format is often seen in user interfaces and forms.
- DD-MM-YYYY: Popular in many European countries, this format is frequently used in official documents and reports.
To implement these formats, you can use the format
method of Moment.js. For instance:
console.log(date.format('MM/DD/YYYY')); // Output: 12/31/2023
console.log(date.format('DD-MM-YYYY')); // Output: 31-12-2023
Moment.js excels in its comprehensive functionality and ease of use, making it a preferred choice for many developers. However, it is important to note some limitations. Moment.js is considered somewhat heavy in terms of package size, which can impact performance in larger projects. Additionally, Moment.js is in maintenance mode, meaning it no longer receives new features, though it still gets critical bug fixes and security updates.
In comparison, libraries like date-fns offer a more modern approach with a modular architecture, allowing developers to import only the functions they need, thus reducing the overall bundle size. Despite this, Moment.js remains a robust and reliable tool for date formatting tasks, especially for legacy codebases and projects where its extensive functionality is required.
Comparing date-fns and Moment.js
When it comes to date formatting in JavaScript, two popular libraries often come into play: date-fns and Moment.js. Both have their unique strengths and weaknesses, making them suitable for different use cases. This section provides an in-depth comparison between these two libraries, focusing on size, functional programming support, and timezone handling capabilities.
Size and Performance
One of the most significant differences between date-fns and Moment.js lies in their sizes and the impact on application performance. Moment.js, while feature-rich, is quite large, with a library size of approximately 67KB minified and gzipped. This size can significantly affect load times, especially for web applications where performance is critical. On the other hand, date-fns is designed to be modular, allowing developers to import only the functions they need. This modularity translates to a much smaller footprint, often around 16KB when using common functionalities. The smaller size of date-fns can lead to faster load times and a more responsive application, making it a preferred choice for performance-sensitive projects.
Functional Programming Paradigm
Date-fns embraces the functional programming paradigm, which can be a significant advantage for developers who prefer this approach. Each function in date-fns is a pure function, meaning it does not mutate the input data but rather returns a new instance with the desired modifications. This immutability aligns well with modern JavaScript practices and promotes safer, more predictable code. In contrast, Moment.js relies on a more object-oriented approach, often mutating the original date object. While this can be convenient, it may introduce side effects and make code harder to debug.
Timezone Handling
Handling timezones is another critical aspect of date manipulation. Moment.js includes comprehensive timezone support through the Moment Timezone extension, allowing developers to parse, manipulate, and display dates in various timezones effortlessly. This feature is particularly useful for applications that need to handle international dates and times. Date-fns, however, does not natively support timezones out of the box. Developers need to rely on additional libraries like timezone-support or date-fns-tz to achieve similar functionality. While this approach can be more modular, it adds complexity to the implementation.
In conclusion, choosing between date-fns and Moment.js depends on the specific requirements of your project. If performance and modularity are your primary concerns, date-fns offers a lightweight and functional approach. However, if you need robust timezone handling and prefer an object-oriented paradigm, Moment.js might be the better choice. Understanding these key differences will help you make an informed decision tailored to your application’s needs.
Best Practices for Date Formatting in JavaScript
Ensuring consistency and reliability in date formatting across web applications is crucial for maintaining a professional and user-friendly interface. One of the foremost practices is to maintain a consistent date format throughout the application. This involves deciding on a standard format early in the development process and applying it uniformly. For example, using the toLocaleDateString()
method in JavaScript can help achieve this. By sticking to a format like ‘YYYY-MM-DD’ or ‘MM-DD-YYYY,’ developers can avoid confusion and provide a seamless user experience.
Another essential practice is locale-specific formatting. Web applications often serve a global audience, and date formats can vary significantly from one locale to another. JavaScript’s Intl.DateTimeFormat
object is particularly useful in this regard, as it allows developers to format dates according to the conventions of different locales. For instance, while the American date format is ‘MM-DD-YYYY,’ many European countries use ‘DD-MM-YYYY.’ Implementing locale-specific formatting ensures that dates are presented in a familiar and comprehensible manner to all users, enhancing the overall accessibility of the application.
The ISO 8601 standard is another critical aspect of best practices in date formatting. This internationally recognized format, which represents dates as ‘YYYY-MM-DD,’ offers several benefits, especially for date storage and APIs. It ensures that dates are unambiguous and easily sortable, which is invaluable for data integrity and interoperability between systems. Utilizing the ISO 8601 format can help developers avoid potential pitfalls related to date parsing and formatting inconsistencies, making it a reliable choice for both internal storage and external API communications.
In summary, adopting these best practices—consistent formatting, locale-specific formatting, and the ISO 8601 standard—can significantly enhance the reliability and user experience of web applications. By implementing these strategies, developers can ensure that their date formatting solutions are robust, intuitive, and internationally compatible.
Conclusion
Mastering date formatting in JavaScript is an essential skill for any web developer, given the significance of correctly handling dates and times in web applications. Throughout this guide, we explored various methods and libraries that facilitate date formatting.
Firstly, we discussed the use of native JavaScript methods. JavaScript’s built-in Date
object provides fundamental functionalities to handle dates and times. Methods like toLocaleDateString()
and toISOString()
are particularly useful for formatting dates in a readable and standardized manner.
Next, we delved into date-fns
, a popular library known for its lightweight and modular approach. date-fns
offers a comprehensive set of functions to manipulate and format dates, simplifying many common tasks. Its functional programming style makes it an excellent choice for modern JavaScript applications.
We also examined Moment.js
, another widely-used library. Although Moment.js is vast and feature-rich, it is important to note that it is no longer in active development. However, it remains a valuable tool for handling complex date and time operations, particularly in legacy projects.
Adopting best practices for date formatting is crucial. Consistency, timezone management, and localization are key factors to consider. Properly formatted dates enhance the user experience and ensure that your application remains reliable and user-friendly across different regions and timezones.
For those looking to deepen their understanding, we encourage exploring additional resources. The official documentation for date-fns and Moment.js provides detailed insights and examples. Additionally, numerous articles and tutorials are available to guide you through advanced techniques for date formatting in JavaScript.
We hope this comprehensive guide has provided you with a solid foundation in date formatting. Continue to experiment and explore the various tools and methods available to enhance your web development projects.
Feel free to Contact us.