Table of Contents
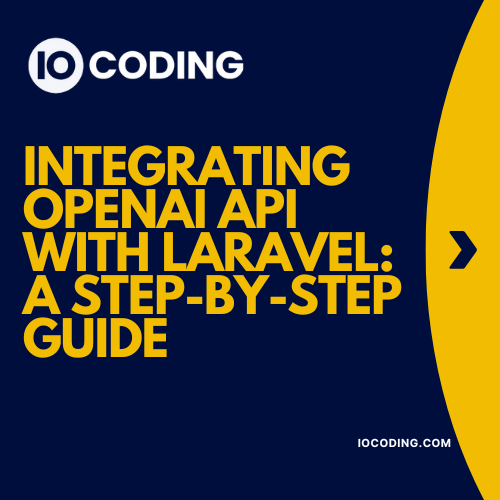
Introduction
Integrating the OpenAI API with Laravel application can unlock a world of possibilities, from advanced text generation to sentiment analysis and more. This guide will walk you through the steps needed to integrate the OpenAI API with Laravel, providing you with practical examples and best practices.
Keywords: Laravel OpenAI integration, OpenAI API Laravel, Laravel text generation, OpenAI PHP SDK
1. Setting Up the Laravel Project
Installing Laravel First, ensure you have Composer installed on your machine. Then, create a new Laravel project:
composer create-project --prefer-dist laravel/laravel openai-laravel
Configuring Environment Next, set up your environment variables. Open the .env
file and configure your database settings and other environment variables.
APP_NAME=OpenAILaravel
APP_ENV=local
APP_KEY=base64:YOUR_APP_KEY
APP_DEBUG=true
APP_URL=http://localhost
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=openai_laravel
DB_USERNAME=root
DB_PASSWORD=
2. Installing Necessary Packages
HTTP Client Laravel provides an in-built HTTP client, which is powered by GuzzleHTTP. Ensure you have Guzzle installed:
composer require guzzlehttp/guzzle
OpenAI PHP SDK You may also need an OpenAI PHP SDK to simplify API calls. If available, install it via Composer:
composer require openai-php/client
3. Creating the OpenAI Service
Service Class Create a service class to handle OpenAI API interactions. Create a new directory Services
and a file OpenAIService.php
:
<?php
namespace App\Services;
use GuzzleHttp\Client;
class OpenAIService
{
protected $client;
public function __construct()
{
$this->client = new Client(['base_uri' => 'https://api.openai.com/v1/']);
}
public function request($method, $endpoint, $params = [])
{
$response = $this->client->request($method, $endpoint, [
'headers' => [
'Authorization' => 'Bearer ' . env('OPENAI_API_KEY'),
'Content-Type' => 'application/json',
],
'json' => $params,
]);
return json_decode($response->getBody(), true);
}
}
API Key Management Store your OpenAI API key securely in the .env
file:
OPENAI_API_KEY=your_openai_api_key
4. Making API Requests
Basic API Request Here’s an example of making a simple API request to OpenAI:
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\Services\OpenAIService;
class OpenAIController extends Controller
{
protected $openAIService;
public function __construct(OpenAIService $openAIService)
{
$this->openAIService = $openAIService;
}
public function generateText(Request input)
{
$prompt = $request->input('prompt');
$response = $this->openAIService->request('POST', 'completions', [
'model' => 'text-davinci-002',
'prompt' => $prompt,
'max_tokens' => 100,
]);
return response()->json($response);
}
}
Advanced Requests To perform more complex tasks, you can adjust the parameters accordingly. For instance, for sentiment analysis, use a different endpoint or modify the parameters.
5. Integrating OpenAI API with Laravel
Controller Setup Add a controller to handle OpenAI interactions:
php artisan make:controller OpenAIController
Routes Define routes in routes/web.php
:
use App\Http\Controllers\OpenAIController;
Route::post('/generate-text', [OpenAIController::class, 'generateText']);
Views Create views to interact with the API. For example, create a form to accept user input in resources/views/generate.blade.php
:
<!DOCTYPE html>
<html>
<head>
<title>Generate Text</title>
</head>
<body>
<form action="/generate-text" method="POST">
@csrf
<textarea name="prompt" placeholder="Enter your prompt here"></textarea>
<button type="submit">Generate</button>
</form>
</body>
</html>
6. Example Use Cases
Text Generation Use OpenAI to generate text based on user input.
Sentiment Analysis Analyze the sentiment of user-provided text.
Chatbot Integration Integrate OpenAI into a chatbot to provide intelligent responses.
7. Testing and Debugging
Testing API Requests Use tools like Postman to test your API requests.
Debugging Common Issues Check for common issues like incorrect API keys or network errors and debug accordingly.
8. Best Practices and Security
API Key Security Never expose your API keys in client-side code.
Rate Limiting and Error Handling Implement rate limiting and proper error handling to manage API limits and handle errors gracefully.
Conclusion
Integrating the OpenAI API with Laravel opens up a myriad of possibilities for enhancing your applications with AI capabilities. By following this guide, you can set up and start using OpenAI’s powerful features in your Laravel projects. For more detailed information, check out the OpenAI API documentation and the Laravel documentation.
For Software Development services, feel free to Contact Us.