Docker is an open-source platform designed to automate the deployment, scaling, and management of applications through containerization. At its core, Docker enables developers to package applications and their dependencies into lightweight, portable containers that can run consistently across various computing environments. This technology has revolutionized the way software is developed, tested, and deployed, offering significant advantages for local development environments.
One of the fundamental concepts in Docker is the container. A container is a standardized unit of software that bundles the application code with its libraries, dependencies, and runtime environment. This ensures that the application behaves the same way regardless of where it’s executed, be it a developer’s local machine, a testing server, or a production environment. This consistency helps in mitigating the common “it works on my machine” problem that often plagues software development projects.
Another key term is the Docker image, which is a read-only template used to create containers. Images are built from a series of layers, each representing a different stage in the construction of the container. These layers can be reused across different images, making the process of building and deploying applications more efficient. Developers can create custom images or use pre-built images from Docker Hub, a repository of Docker images shared by the community.
Docker’s architecture is based on a client-server model. The Docker client communicates with the Docker daemon, which does the heavy lifting of building, running, and managing containers. This separation of concerns allows for a more modular and flexible development workflow. Docker Compose, another tool within the Docker ecosystem, further enhances this workflow by enabling the definition and management of multi-container applications.
The benefits of using Docker for local development are manifold. It simplifies the setup of development environments, ensuring that all team members work in identical conditions. Docker also accelerates the onboarding process for new developers, as they can quickly spin up the necessary environment with minimal configuration. Additionally, Docker’s isolation capabilities allow developers to run multiple project environments on a single machine without conflicts.
Overall, Docker offers a robust and streamlined approach to managing application environments, facilitating a more efficient and reliable development process. With a solid understanding of Docker’s fundamental concepts and functionalities, developers can leverage this powerful tool to enhance their local development workflows.
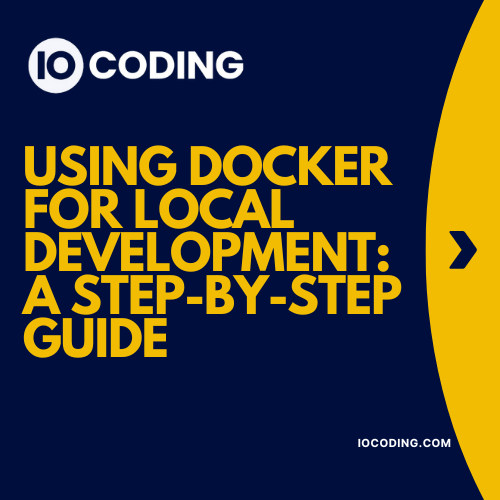
Table of Contents
Installing Docker
Before you can leverage Docker for local development, the first crucial step is to install Docker on your operating system. Docker is available on Windows, macOS, and Linux, and each OS has specific installation steps and prerequisites that need to be followed. This section will guide you through the detailed process for each OS, ensuring that by the end, Docker will be successfully installed and ready for use.
Windows
For Windows users, Docker offers Docker Desktop, a user-friendly application that simplifies the installation process. To begin, navigate to the Docker Desktop for Windows page and download the installer.
1. Run the downloaded installer and follow the on-screen instructions.
2. Ensure that the “Enable WSL 2” checkbox is selected to use the Windows Subsystem for Linux 2, which significantly improves Docker’s performance on Windows.
3. After the installation completes, the Docker Desktop application will launch automatically. You may be prompted to sign in or create a Docker Hub account.
4. Verify the installation by opening a command prompt and running docker --version
. This command should return the installed Docker version.
macOS
macOS users can install Docker Desktop for Mac, which integrates seamlessly with the macOS operating system. Start by visiting the Docker Desktop for Mac page and downloading the installer.
1. Open the downloaded .dmg file and drag the Docker icon to your Applications folder.
2. Launch Docker from the Applications folder. You may need to provide your system password to complete the installation.
3. Once Docker is running, an icon will appear in the menu bar. Click the icon to access Docker’s settings and preferences.
4. Verify the installation by opening a terminal and running docker --version
, which should display the installed Docker version.
Linux
For Linux users, Docker can be installed via the package management system specific to your distribution. Here, we will cover the installation process for Ubuntu:
1. Update your existing list of packages: sudo apt update
2. Install the necessary prerequisites: sudo apt install apt-transport-https ca-certificates curl software-properties-common
3. Add Docker’s official GPG key: curl -fsSL https://download.docker.com/linux/ubuntu/gpg | sudo apt-key add -
4. Add the Docker repository to APT sources: sudo add-apt-repository "deb [arch=amd64] https://download.docker.com/linux/ubuntu $(lsb_release -cs) stable"
5. Update the package database again: sudo apt update
6. Finally, install Docker: sudo apt install docker-ce
7. Verify the installation by running docker --version
in the terminal, which should return the installed Docker version.
By following these instructions, you should have Docker installed on your local machine, regardless of your operating system. This will set the foundation for utilizing Docker in your local development environment.
Setting Up a Development Environment with Docker
Setting up a development environment using Docker begins with the creation of a project directory. This directory will house all the necessary files for your Dockerized environment. Start by creating a new directory for your project on your local machine using the command:
mkdir my-docker-project
Navigate into this directory:
cd my-docker-project
Next, you will need to create a Dockerfile, which is a script containing a series of instructions on how to build a Docker image for your development environment. Within your project directory, create a new file named Dockerfile
:
touch Dockerfile
Open the Dockerfile
in your preferred text editor and define the base image and environment setup. For example, if you’re setting up a Node.js environment, your Dockerfile might look like this:
FROM node:14
WORKDIR /usr/src/app
COPY package*.json ./
RUN npm install
COPY . .
EXPOSE 3000
CMD ["node", "app.js"]
This Dockerfile uses the Node.js 14 image as the base, sets the working directory inside the container, copies project dependencies, installs them, and finally exposes port 3000 where the Node.js application will run.
With your Dockerfile defined, the next step is to build the Docker image. From the root of your project directory, run the following command:
docker build -t my-node-app .
This command tells Docker to build an image using the Dockerfile in the current directory (denoted by the .
), and tag it as my-node-app
.
Once the image is built, you can run a container from this image using the command:
docker run -p 3000:3000 my-node-app
This command maps port 3000 on your local machine to port 3000 in the container, allowing you to access the running application at http://localhost:3000
.
By following these steps, Docker standardizes and streamlines your development workflow, ensuring consistency across different environments and simplifying the setup process.
Creating and Managing Containers
Building Docker images and running containers are fundamental aspects of utilizing Docker for local development. These tasks are accomplished using a series of Docker commands, starting with the creation of Dockerfiles. A Dockerfile is a script containing a series of instructions on how to build a Docker image. To create an image, navigate to the directory containing your Dockerfile and use the command:
docker build -t your_image_name .
Here, the -t flag tags the image with a name for easier reference. Once the image is built, it can be run as a container using the following command:
docker run -d --name your_container_name your_image_name
The -d flag runs the container in detached mode, allowing it to run in the background. The –name flag assigns a name to the container for simpler management. To list all running containers, use:
docker ps
Stopping a running container is straightforward with the docker stop
command, followed by the container’s name or ID:
docker stop your_container_name
To restart a stopped container, the docker start
command is used:
docker start your_container_name
Managing the lifecycle of containers involves additional commands. For instance, to remove a stopped container, the docker rm
command is used:
docker rm your_container_name
Similarly, Docker images can be managed using commands such as docker images
to list all images and docker rmi
to remove an image:
docker rmi your_image_name
These basic commands are essential for maintaining a clean and efficient Docker environment. Regularly cleaning up unused containers and images can help in optimizing disk space and system performance. By mastering these Docker commands, developers can streamline their local development workflows and ensure their Docker environments remain organized and efficient.
Docker Compose for Multi-Container Applications
Docker Compose is a powerful tool that simplifies the orchestration and management of multi-container Docker applications. By using Docker Compose, developers can define and run applications consisting of multiple containers using a single configuration file. This eliminates the need to manage each container individually, thus streamlining the development process considerably.
The primary benefit of Docker Compose lies in its ability to manage complex applications with ease. It allows developers to define services, networks, and volumes in a single docker-compose.yml
file. This file serves as a blueprint for the entire application, detailing how each container should be built, configured, and interconnected. This approach not only enhances consistency across development environments but also simplifies deployment and scaling operations.
To start with Docker Compose, one must first create a docker-compose.yml
file in the root directory of the project. This file uses YAML syntax to define the services that make up the application. For example, a simple web application might consist of a web server and a database. The following is a basic example of a docker-compose.yml
file:
version: '3'
services:
web:
image: 'nginx:latest'
ports:
- '80:80'
db:
image: 'mysql:latest'
environment:
MYSQL_ROOT_PASSWORD: 'example'
In this example, the web
service uses the latest Nginx image and maps port 80 on the host to port 80 on the container. The db
service uses the latest MySQL image and sets an environment variable for the MySQL root password. This simple setup demonstrates how easy it is to define and run a multi-container application using Docker Compose.
Once the docker-compose.yml
file is ready, the application can be started with a single command:
docker-compose up
This command reads the docker-compose.yml
file, builds the specified images if necessary, creates the containers, and starts the services. To stop the application, use the following command:
docker-compose down
By leveraging Docker Compose, developers can efficiently manage and scale complex applications involving multiple interconnected containers, ultimately enhancing productivity and consistency across different environments.
Volume Management and Persistent Data
In the realm of Docker-based local development, the effective management of volumes is imperative for ensuring data persistence across container lifecycles. Docker volumes serve as a fundamental mechanism for storing data that needs to be kept intact even when containers are stopped or recreated. By leveraging volumes, developers can separate the data from the container’s filesystem, thereby safeguarding it from being lost during container restarts or rebuilds.
To create a Docker volume, you can utilize the following command:
docker volume create my_volume
Once created, the volume can be integrated into a container using the -v
flag. For instance:
docker run -d -v my_volume:/data my_image
In this example, the volume my_volume
is mounted to the /data
directory within the container, ensuring that any data written to this directory persists outside the container’s lifecycle.
It is essential to understand the distinction between volumes and bind mounts. While both can be used for data persistence, volumes are managed by Docker and are typically stored in Docker’s designated area on the host filesystem, making them more portable and easier to manage. Bind mounts, on the other hand, link specific paths on the host to paths in the container, providing more control but requiring careful handling to avoid potential data integrity issues.
Best practices for volume management include regularly backing up volumes, monitoring storage usage, and organizing volumes logically according to application needs. To back up a volume, you can use the docker run
command to create a new container that mounts the volume and copies its contents to a backup location:
docker run --rm -v my_volume:/data -v $(pwd):/backup busybox tar cvf /backup/backup.tar /data
This command creates a tarball of the volume’s contents and stores it in the current directory. Regular monitoring of volume storage usage can be achieved through Docker’s built-in commands, such as docker system df
, which provides an overview of disk usage by Docker objects, including volumes.
In summary, by adhering to these best practices and understanding the functionality and importance of Docker volumes, developers can ensure data persistence and integrity, thereby enhancing the robustness of their local development environments.
Networking with Docker
Docker networking is a vital component for enabling containers to communicate with each other and with external systems. Docker manages networks using a variety of drivers, each tailored to different networking needs. The default network driver, called ‘bridge’, is ideal for standalone containers. When a new container is created, Docker automatically connects it to the default ‘bridge’ network, allowing basic communication and isolation from other containers and the host system.
For more complex networking requirements, Docker allows the creation of custom networks. Custom networks provide enhanced functionality such as automatic DNS resolution, which simplifies container linking and name resolution. To create a custom network, use the following command:
docker network create <network_name>
Once a custom network is created, containers can be connected to it at runtime using the --network
flag. For example:
docker run -d --name my_container --network my_network my_image
Linking containers can be achieved by placing them on the same network. This allows them to communicate using their container names as hostnames. For instance, if you have two containers, ‘web’ and ‘db’, both connected to a custom network named ‘my_network‘, the ‘web’ container can access the ‘db’ container using the hostname ‘db’.
Moreover, Docker supports advanced network settings through the use of network drivers like ‘overlay’ for multi-host networking and ‘macvlan’ for direct integration with physical network interfaces. These drivers can be configured to meet specific networking requirements, enhancing the flexibility and scalability of containerized applications.
To manage network settings effectively, Docker provides several commands such as docker network ls
to list available networks, docker network inspect
to view network details, and docker network rm
to remove unused networks.
By understanding how to create, configure, and manage Docker networks, developers can ensure that their containerized applications communicate efficiently and securely, paving the way for robust and scalable local development environments.
Best Practices for Using Docker in Development
When leveraging Docker for local development, adhering to best practices can significantly enhance performance, security, and overall productivity. Implementing these strategies ensures that your development environment remains efficient and secure.
Firstly, performance optimization is crucial. To achieve optimal performance, consider using lightweight base images. Smaller images can drastically reduce build times and resource consumption. Additionally, take advantage of Docker’s multi-stage builds to keep image sizes minimal and avoid unnecessary files in the final image. Regularly cleaning up unused Docker images, containers, and volumes is another effective way to maintain a lean development environment.
Secondly, security should not be overlooked. Always pull images from trusted sources, and verify the integrity of these images through checksums. Using Docker’s built-in security features, such as user namespaces and seccomp profiles, can add an extra layer of protection. Implementing least privilege principles by running containers with the minimal required permissions helps mitigate potential vulnerabilities. Keeping Docker and its dependencies up-to-date ensures you are protected against the latest security threats.
Efficient workflow strategies also play a significant role in optimizing Docker use. Docker Compose can be a powerful tool for managing multi-container applications, simplifying the process of configuring and running services. Utilize Docker volumes for persistent data storage, which can be particularly useful during development iterations. Moreover, leveraging Docker’s caching mechanisms can speed up the build process and reduce redundant tasks.
Another best practice is to maintain clear and concise Dockerfiles. Commenting on each instruction and organizing related commands can make Dockerfiles more readable and easier to maintain. Version control for Dockerfiles can track changes and facilitate collaboration among team members.
By following these best practices, developers can harness the full potential of Docker in their development environments. The result is a streamlined, secure, and efficient workflow that enhances productivity and ensures a reliable development process.
Conclusion – Docker for Local Development
In this guide, we have explored the fundamental aspects of using Docker for local development. From setting up Docker on your machine to creating and managing containers, each step has been designed to streamline your development process. Docker’s ability to replicate production environments locally ensures consistency and reliability, significantly reducing the “it works on my machine” problem.
By integrating Docker into your local development workflow, you can enjoy numerous benefits such as simplified dependency management, enhanced collaboration among team members, and the ability to easily scale applications. Docker containers provide an isolated environment, ensuring that your applications run smoothly regardless of the underlying system configurations.
We encourage you to start experimenting with Docker and leverage its powerful features to improve your development practices. Whether you are working on a small project or a large-scale application, Docker can help you achieve a more efficient and organized workflow. To further enhance your understanding, consider exploring additional resources, such as the official Docker documentation, community forums, and online tutorials. These resources can offer valuable insights and troubleshooting tips as you continue your journey with Docker.
Embracing Docker for local development not only boosts productivity but also prepares you for modern DevOps practices. As you become more comfortable with Docker, you will find it easier to transition to container orchestration tools like Kubernetes, further enhancing your development and deployment processes. We hope this guide has provided you with the necessary knowledge and motivation to start using Docker in your local development environment. Happy coding!
Feel free to Contact us.